How to Optimize React Native Apps for Better Performance and Battery Life
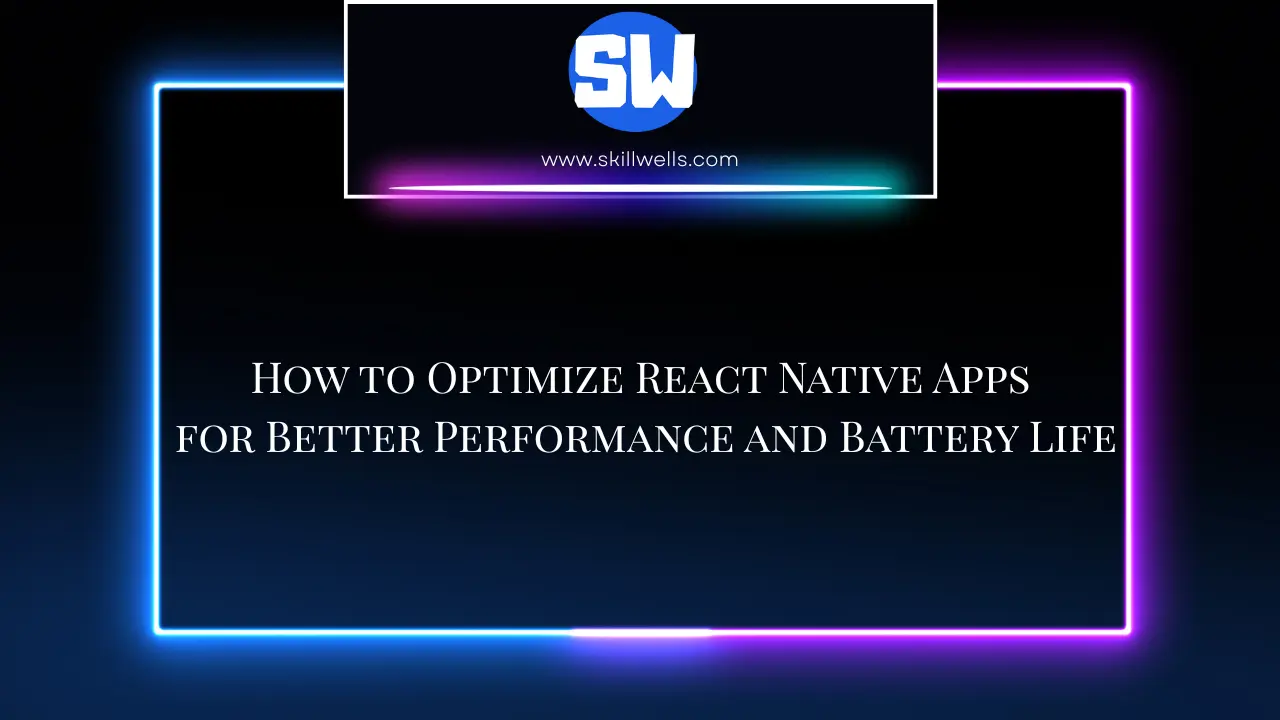
React Native is a powerful framework for building cross-platform mobile applications. While performance optimization and battery life are important for mobile users, in this post, we describe the best ways to achieve these goals.
If you are new to React Native, you must first expand your skills to become an app developer for Android and iOS systems. We suggest you study the React Native Masterclass and then return and Optimize React Native Apps as much as possible!
1. Use the latest version of React Native
Updated versions of React Native often contain operation improvements and bug fixes, so keep your application updated to take advantage of these developments. To update React Native, use the code:
Npx react-native upgrade |
2. Decrease unnecessary re-renders
Unnecessary re-renders can decrease program speed. To prevent these problems, do one of the items below:
- Use React. Memo for functional components that don’t need re-renders.
- Use of PureComponent instead of Component in class-based components.
- Use of useCallback and useMemo to save functions and values.
To understand this method better, pay attention to example:
Const MyComponent = React.memo(({data}) → { Return {data}; |
3. Image optimization
Big images can slow down program speed and increase battery usage. To optimize photos, it’s better to use WebP format instead of PNG or JPG to compress them more. Change image sizes appropriate to screen resolution and use CDN to download. The use of react-native-fast-image as an example:
Import FastImage from ‘react-native-fast-image’; Style={{width: 100, height: 100}} Source={{uri: ‘https://your-cdn.com/image.webp’}} resizeMode={FastImage.resizeMode.contain} /> |
4. Reducing size of JavaScript bundle
Big javascript bundles increase startup time. To optimize them, delete unnecessary dependencies. Use Hermes motors (light javascript motor) for android and enable code splitting and lazy loading.
To enable Hermes, edit the android/app/build.gradle file:
Project.ext.react = [ enableHermes: true // Add this line ] |
5. State management optimization
Inappropriate State Management updates result in performance loss. To optimize, avoid unnecessary use-state updates. Also, use optimized Context API or Redux and, for simple state management, use Zustand or Recoil instead of Redux.
6. Reduce unnecessary requests for servers
Large amounts of API requests can slow down programs and increase battery usage. To optimize it, use caching such as AsyncStorage or React Query. For input searches, use Debouncing to avoid sending large amounts of requests. And for real-time updates, use WebSocket instead of polling.
7. Use of native modules for heavy duties
To do heavy duties such as image processing or animations, use native modules to have better performance. For example, use react-native-reanimated for animations. Use react-native-gesture-handler for smother gestures.
8. Optimizing lists with FlatList
To preview long lists, don’t use ScrollView; instead use FlatList with optimized settings. For example:
Data={data} renderItem={({item}) → {item.name}} keyExtractor={(item) → item.id.toString()} initialNumToRender={10} // Reduce initial load maxToRenderPerBatch={5} // Optimize rendering removeClippedSubviews={true} // Improve performance /> |
9. Performance optimization and supervision
There are some tools for program operation supervision. React Native Profiler is for troubleshooting operational problems. Flipper is used for checking network requests, logs and other items and Perf Monitor is used for developer settings.
10. Optimizing battery usage to optimize React Native Apps
To reduce battery usage, decrease background services and unnecessary timers. Also, use GPS when it is necessary. Decrease the use of animation when the battery is low and finally avoid too much polling and background tasks.
Conclusion
Optimizing operation and battery usage of React Native programs provides a better user experience. By reducing unnecessary re-renders, optimizing images, reducing network requests and the use of native modules, you can build fast and low-consuming programs. To see the result, do all the techniques described.